Food delivery business code forms the backbone of modern food ordering and delivery services, a rapidly evolving industry. This code encompasses everything from user-friendly interfaces and efficient order management to secure payment processing and scalable backend infrastructure. Understanding the intricacies of this code is crucial for anyone looking to build, maintain, or improve a food delivery platform.
This exploration delves into the essential components, architectural considerations, and best practices that define robust and successful food delivery business code. We’ll examine core modules like user authentication, restaurant management, and payment integration, alongside critical aspects such as UI/UX design, database schema, order management, and scaling strategies. From the initial concept to deployment and beyond, this guide offers a comprehensive overview of the key elements involved.
Core Components of a Food Delivery Business Code
A food delivery business code is a complex system designed to manage various aspects of the delivery process, from user interaction to payment processing. This intricate system requires several core modules to function efficiently and provide a seamless experience for users, restaurants, and delivery personnel. These modules must be robust, secure, and scalable to handle the demands of a growing business.
User Authentication
User authentication is a fundamental component that secures access to the platform. This involves verifying the identity of users, restaurants, and delivery personnel. The system must be designed to protect sensitive information and prevent unauthorized access.The following functions and classes typically handle user registration and login:
- Registration: A `UserRegistration` class or function handles the creation of new user accounts. This involves:
- Collecting user information such as name, email, phone number, and password.
- Validating the provided information to ensure it meets the required criteria (e.g., password strength, email format).
- Hashing the password using a secure algorithm (e.g., bcrypt, Argon2) before storing it in the database.
- Storing user data in a database.
- Sending a confirmation email or SMS for verification.
- Login: A `UserLogin` class or function handles the authentication process. This involves:
- Retrieving the user’s credentials (email/username and password).
- Validating the credentials against the stored data in the database.
- Comparing the entered password with the hashed password using the same hashing algorithm.
- Generating a session token or JWT (JSON Web Token) upon successful authentication.
- Managing user sessions.
- Password Reset: Functionality for users to reset forgotten passwords, usually including a secure mechanism to send password reset links to registered email addresses.
- Role-Based Access Control (RBAC): Implementing RBAC to define different roles (e.g., customer, restaurant admin, delivery personnel, admin) and their respective permissions within the system.
Restaurant Management
Restaurant management is the module responsible for managing restaurant information, menus, and operational details. It allows restaurants to register, update their profiles, and manage their offerings.Data structures used to store restaurant information are critical for efficiency and organization. Here are some key data structures:
- Restaurant Data Structure: This typically uses a database table or a class in the code to store core restaurant details:
- Restaurant ID (unique identifier).
- Restaurant Name.
- Address (including street, city, zip code, and coordinates).
- Contact Information (phone number, email).
- Operating Hours (defined as a schedule).
- Cuisine Type (e.g., Italian, Mexican).
- Restaurant Images (logo, photos).
- Delivery Zone (geographical area).
- Minimum Order Amount.
- Delivery Fee.
- Menu Item Data Structure: Each menu item requires a structure that includes:
- Menu Item ID (unique identifier).
- Restaurant ID (foreign key linking to the restaurant).
- Item Name.
- Description.
- Price.
- Category (e.g., Appetizers, Main Courses, Desserts).
- Images.
- Ingredients.
- Availability (e.g., available, out of stock).
- Operating Hours Data Structure: The operating hours are commonly stored as a schedule, allowing for different hours on different days:
- Day of the Week.
- Opening Time.
- Closing Time.
Order Processing
Order processing is the module that handles the complete lifecycle of an order, from placement to delivery. It includes order placement, assignment to delivery personnel, and real-time tracking.Algorithms that manage order placement, assignment, and tracking are crucial for efficiency:
- Order Placement Algorithm: This algorithm manages the order creation process:
- Receives order details from the user, including selected items, quantities, and delivery address.
- Calculates the order total, including taxes and delivery fees.
- Validates order details (e.g., checking item availability, ensuring the restaurant is open).
- Creates an order record in the database.
- Sends a confirmation to the user and the restaurant.
- Delivery Personnel Assignment Algorithm: This algorithm assigns the order to a delivery person:
- Proximity-Based Assignment: Orders are assigned to the delivery person closest to the restaurant or the customer.
- Availability Check: Checks the availability and current status of delivery personnel (e.g., available, busy, offline).
- Performance-Based Assignment: Considers delivery personnel ratings, order completion rates, and historical performance.
- Load Balancing: Distributes orders evenly among delivery personnel.
- Dynamic Pricing: Adjusts delivery fees based on demand and delivery personnel availability.
- Real-Time Tracking Algorithm: Provides real-time updates on order status:
- Tracks the delivery person’s location using GPS data.
- Updates the order status (e.g., order placed, preparing, en route, delivered).
- Displays the delivery person’s location on a map.
- Provides estimated time of arrival (ETA) based on real-time traffic data.
Payment Integration
Payment integration is a critical module for handling financial transactions securely. It allows users to pay for their orders and ensures that restaurants and delivery personnel are compensated.Methods for integrating payment gateways and handling financial transactions securely include:
- Payment Gateway Integration: Integrating with payment gateways such as Stripe, PayPal, or Braintree to process payments.
- API Integration: Using the payment gateway’s APIs to handle payment processing.
- Tokenization: Storing sensitive card information securely using tokenization.
- Subscription Management: Handling recurring payments for subscriptions or memberships.
- Secure Transactions: Ensuring secure transactions to protect user data and prevent fraud.
- SSL/TLS Encryption: Using SSL/TLS encryption to encrypt all data transmitted between the user’s device and the server.
- PCI DSS Compliance: Adhering to PCI DSS (Payment Card Industry Data Security Standard) requirements for handling credit card information.
- Fraud Detection: Implementing fraud detection mechanisms to identify and prevent fraudulent transactions.
- Financial Reporting: Generating financial reports for tracking revenue, expenses, and payouts.
- Transaction Logging: Logging all financial transactions for auditing purposes.
- Revenue Analysis: Analyzing revenue data to identify trends and insights.
- Payout Management: Managing payouts to restaurants and delivery personnel.
User Interface (UI) and User Experience (UX) Considerations: Food Delivery Business Code
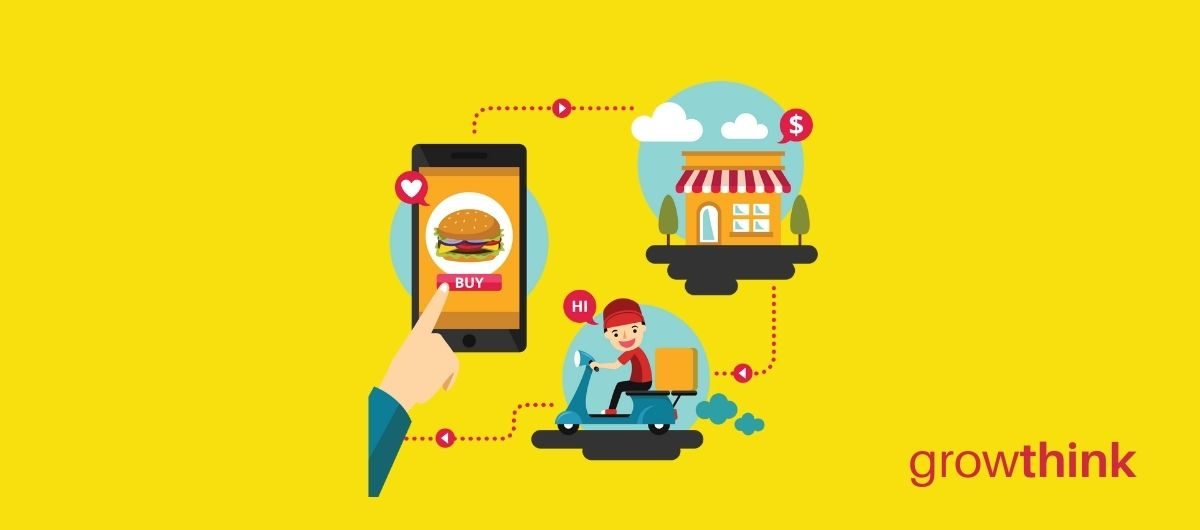
Designing a compelling user interface (UI) and ensuring a positive user experience (UX) are paramount for the success of a food delivery application. This involves creating an intuitive and visually appealing interface that facilitates easy navigation, order placement, and overall user satisfaction. It also requires careful consideration of user behavior, feedback mechanisms, and responsiveness across different devices.The focus is on designing the user interface flow, key UI elements, restaurant profile components, handling user input, and ensuring responsiveness.
These elements collectively contribute to a seamless and enjoyable experience for both customers and restaurants.
User Interface Flow for a Food Delivery App
The user interface flow should guide users through a series of logical steps, from app launch to order confirmation. This flow ensures a smooth and efficient process, minimizing user frustration and maximizing order completion rates.The following Artikels the typical steps in a food delivery app’s user interface flow:
- App Launch: The user opens the application. The initial screen typically displays a loading screen with the app’s logo and branding, followed by the main interface.
- Location Services: The app prompts the user to enable location services, either automatically detecting the user’s location or allowing manual input. This is crucial for showing nearby restaurants.
- Restaurant Browsing: The user browses a list of restaurants, often categorized by cuisine, ratings, distance, or special offers. Restaurants can be displayed in a list view, map view, or both.
- Restaurant Selection: The user selects a restaurant to view its profile, including the menu, ratings, reviews, and other relevant information.
- Menu Browsing and Item Selection: The user browses the restaurant’s menu, selecting desired items and customizing them (e.g., adding toppings, specifying quantities, or adding special instructions).
- Order Review: The user reviews the selected items in their order, including quantities, customizations, and subtotal.
- Address and Payment Information: The user enters or selects a delivery address and chooses a payment method (e.g., credit card, mobile payment).
- Order Confirmation: The user confirms the order. The app displays an order summary, including the estimated delivery time and order total.
- Order Tracking: The user can track the order’s progress, including the restaurant preparing the food, the driver picking up the order, and the driver’s location on a map.
- Order Delivery: The order is delivered to the user’s specified address.
- Order Completion and Feedback: The user receives the order, and the app prompts them to rate the restaurant and driver, providing feedback on their experience.
Key UI Elements for Customer and Restaurant Interfaces
The design of key UI elements is crucial for the usability and effectiveness of the app. Distinct elements are necessary for both the customer and restaurant interfaces, each tailored to their specific needs and interactions.The following lists detail the key UI elements:
- Customer Interface:
- Search Bar: Allows users to search for restaurants or specific menu items.
- Restaurant Listings: Displays restaurants with their names, ratings, estimated delivery times, and distance.
- Menu Display: Presents menu items with images, descriptions, and prices.
- Order Summary: Shows the selected items, quantities, and subtotal.
- Address Input/Selection: Enables users to input or select their delivery address.
- Payment Options: Displays available payment methods.
- Order Tracking Map: Shows the driver’s location in real-time.
- Ratings and Reviews: Allows users to rate restaurants and drivers and leave reviews.
- Restaurant Interface:
- Order Management Dashboard: Displays incoming orders, order statuses, and order details.
- Menu Management: Allows restaurants to add, edit, and remove menu items.
- Order Confirmation/Rejection Buttons: Enables restaurants to accept or reject incoming orders.
- Order Preparation Status Updates: Allows restaurants to update the status of an order (e.g., preparing, ready for pickup).
- Sales Reports and Analytics: Provides restaurants with data on sales, popular items, and customer behavior.
- Messaging with Customer/Driver: Facilitates communication with customers and delivery drivers.
Components of a Restaurant Profile Page
The restaurant profile page is a critical component of the user interface, as it provides essential information and allows users to interact with the restaurant’s menu. The organization of the components must be intuitive and visually appealing to enhance user engagement.The following Artikels the key components:
- Restaurant Information: This section includes the restaurant’s name, logo, address, contact information, operating hours, and average customer rating.
- Menu Presentation: The menu should be clearly organized, with categories (e.g., appetizers, entrees, desserts) and subcategories. Each menu item should include:
- Item Name: The name of the dish.
- Description: A brief description of the dish, including ingredients.
- Price: The price of the item.
- Image: A high-quality image of the dish.
- Customization Options: Options for the user to customize the dish, such as adding toppings, selecting sizes, or specifying cooking preferences.
- Order Customization: Allows users to specify their preferences for each menu item. This includes:
- Quantity Selection: The ability to select the number of servings.
- Add-ons and Modifications: The ability to add or remove ingredients.
- Special Instructions: A text field for the user to provide specific instructions to the restaurant.
- Reviews and Ratings: Displays customer reviews and ratings.
Methods for Handling User Input and Feedback
Handling user input effectively, including error handling and data validation, is crucial for creating a robust and user-friendly application. This ensures that the app functions correctly and provides a positive experience, even when users make mistakes or encounter issues.The following illustrates methods for handling user input and feedback:
- Data Validation:
- Input Field Validation: Validate user input in real-time to prevent errors. For example, check for valid email addresses, phone numbers, and delivery addresses.
- Form Validation: Validate entire forms before submission, providing clear error messages for any missing or incorrect fields.
- Example: Implement regular expressions to validate email addresses (e.g., `^[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]2,$`) and phone numbers.
- Error Handling:
- Clear Error Messages: Display user-friendly error messages that explain what went wrong and how to correct it.
- Example: If a user enters an invalid credit card number, display a message like “Invalid credit card number. Please check and try again.”
- Server-Side Error Handling: Handle server-side errors gracefully, such as network issues or database errors, and display appropriate messages to the user.
- Feedback Mechanisms:
- Progress Indicators: Use progress bars or spinners to indicate when the app is loading or processing a request.
- Success Messages: Display confirmation messages after successful actions, such as order placement or profile updates.
- Example: After a user places an order, display a message like “Order placed successfully! You will receive an email confirmation shortly.”
Design of a Responsive UI
Designing a responsive UI is essential for ensuring that the food delivery app functions and looks great on various screen sizes and device types. This includes smartphones, tablets, and desktops.The following are key considerations for creating a responsive UI:
- Flexible Layouts: Use flexible grids and layouts that adapt to different screen sizes.
- Media Queries: Utilize CSS media queries to apply different styles based on the screen size and device orientation.
Example:
@media (max-width: 768px) /* Styles for mobile devices
-/ - Responsive Images: Use responsive images that scale appropriately to different screen resolutions. Consider using the `srcset` attribute in the `
` tag.
Example:
<img src="image-small.jpg" srcset="image-small.jpg 480w, image-medium.jpg 768w, image-large.jpg 1024w" sizes="(max-width: 480px) 100vw, (max-width: 768px) 50vw, 33vw" alt="Description of the image">
- Touch-Friendly Design: Ensure that UI elements are touch-friendly, with sufficient spacing between interactive elements.
- Testing: Thoroughly test the app on various devices and screen sizes to ensure that it functions correctly and looks good. Use browser developer tools and device emulators to test different screen sizes.
Backend Architecture and Database Design
The backend architecture forms the backbone of a food delivery business, responsible for handling data storage, processing requests, and ensuring smooth operation. This section delves into the critical aspects of designing a robust and scalable backend, focusing on database schema, database type selection, API endpoints, and data security measures. A well-designed backend is crucial for providing a seamless and efficient experience for users, restaurants, and delivery personnel.
The architecture’s effectiveness directly influences the system’s ability to handle a large volume of orders, manage complex operations, and provide real-time updates, ultimately impacting user satisfaction and business success.
Database Schema for Storing Data
The database schema defines how data is organized and stored within the system. It’s crucial for efficient data retrieval, storage, and management. The following tables represent the core components of the food delivery business and their respective fields:
- Users Table: Stores user-related information.
- user_id (INT, Primary Key, Auto-increment): Unique identifier for each user.
- first_name (VARCHAR): User’s first name.
- last_name (VARCHAR): User’s last name.
- email (VARCHAR, Unique): User’s email address.
- password (VARCHAR): Hashed password for user authentication.
- phone_number (VARCHAR): User’s phone number.
- address (TEXT): User’s delivery address.
- created_at (TIMESTAMP): Timestamp indicating when the user account was created.
- updated_at (TIMESTAMP): Timestamp indicating the last time the user account was updated.
- Restaurants Table: Stores restaurant details.
- restaurant_id (INT, Primary Key, Auto-increment): Unique identifier for each restaurant.
- restaurant_name (VARCHAR): Restaurant’s name.
- description (TEXT): Restaurant’s description.
- cuisine_type (VARCHAR): Type of cuisine offered (e.g., Italian, Chinese).
- address (TEXT): Restaurant’s address.
- latitude (DECIMAL): Restaurant’s latitude coordinate.
- longitude (DECIMAL): Restaurant’s longitude coordinate.
- phone_number (VARCHAR): Restaurant’s phone number.
- opening_hours (TEXT): Restaurant’s opening hours (e.g., “10:00-22:00”).
- image_url (VARCHAR): URL of the restaurant’s image.
- created_at (TIMESTAMP): Timestamp indicating when the restaurant record was created.
- updated_at (TIMESTAMP): Timestamp indicating the last time the restaurant record was updated.
- Orders Table: Stores order information.
- order_id (INT, Primary Key, Auto-increment): Unique identifier for each order.
- user_id (INT, Foreign Key referencing Users table): ID of the user who placed the order.
- restaurant_id (INT, Foreign Key referencing Restaurants table): ID of the restaurant the order is from.
- delivery_personnel_id (INT, Foreign Key referencing DeliveryPersonnel table, Nullable): ID of the delivery person assigned to the order.
- order_status (ENUM): Order status (e.g., “pending”, “confirmed”, “preparing”, “out for delivery”, “delivered”, “cancelled”).
- order_date (DATE): Date the order was placed.
- order_time (TIME): Time the order was placed.
- delivery_address (TEXT): Delivery address for the order.
- total_amount (DECIMAL): Total amount of the order.
- payment_method (VARCHAR): Payment method used (e.g., “credit card”, “cash”).
- created_at (TIMESTAMP): Timestamp indicating when the order was created.
- updated_at (TIMESTAMP): Timestamp indicating the last time the order was updated.
- Order Items Table: Stores details of items within an order.
- order_item_id (INT, Primary Key, Auto-increment): Unique identifier for each order item.
- order_id (INT, Foreign Key referencing Orders table): ID of the order the item belongs to.
- menu_item_id (INT, Foreign Key referencing MenuItems table): ID of the menu item.
- quantity (INT): Quantity of the item ordered.
- item_price (DECIMAL): Price of the item.
- created_at (TIMESTAMP): Timestamp indicating when the order item was created.
- updated_at (TIMESTAMP): Timestamp indicating the last time the order item was updated.
- Menu Items Table: Stores information about menu items.
- menu_item_id (INT, Primary Key, Auto-increment): Unique identifier for each menu item.
- restaurant_id (INT, Foreign Key referencing Restaurants table): ID of the restaurant the menu item belongs to.
- item_name (VARCHAR): Name of the menu item.
- description (TEXT): Description of the menu item.
- price (DECIMAL): Price of the menu item.
- image_url (VARCHAR): URL of the menu item’s image.
- created_at (TIMESTAMP): Timestamp indicating when the menu item was created.
- updated_at (TIMESTAMP): Timestamp indicating the last time the menu item was updated.
- DeliveryPersonnel Table: Stores delivery personnel information.
- delivery_personnel_id (INT, Primary Key, Auto-increment): Unique identifier for each delivery personnel.
- first_name (VARCHAR): Delivery personnel’s first name.
- last_name (VARCHAR): Delivery personnel’s last name.
- email (VARCHAR, Unique): Delivery personnel’s email address.
- phone_number (VARCHAR): Delivery personnel’s phone number.
- vehicle_type (VARCHAR): Type of vehicle (e.g., “bike”, “scooter”, “car”).
- availability_status (ENUM): Delivery personnel’s availability status (e.g., “available”, “busy”, “offline”).
- latitude (DECIMAL): Delivery personnel’s current latitude coordinate.
- longitude (DECIMAL): Delivery personnel’s current longitude coordinate.
- created_at (TIMESTAMP): Timestamp indicating when the delivery personnel record was created.
- updated_at (TIMESTAMP): Timestamp indicating the last time the delivery personnel record was updated.
Advantages and Disadvantages of Database Types
Choosing the right database type is crucial for the performance, scalability, and maintainability of the food delivery platform. Relational databases and NoSQL databases each offer distinct advantages and disadvantages, making the selection process a critical architectural decision. Here’s a comparison:
- Relational Databases (e.g., MySQL, PostgreSQL):
- Advantages:
- ACID properties: Ensures data integrity through atomicity, consistency, isolation, and durability. This is particularly important for financial transactions and order processing.
- Data consistency: Enforces strict data schemas, ensuring data accuracy and reliability.
- Mature technology: Well-established and supported, with a wealth of tools and expertise available.
- Joins and complex queries: Enables complex queries and relationships between data.
- Disadvantages:
- Scalability challenges: Scaling can be more complex compared to NoSQL databases, especially for handling massive amounts of data.
- Rigid schema: Changes to the schema can be time-consuming and require downtime.
- Performance bottlenecks: Complex joins and queries can lead to performance issues under high load.
- NoSQL Databases (e.g., MongoDB, Cassandra):
- Advantages:
- Scalability: Designed to scale horizontally, making it easier to handle large volumes of data and traffic.
- Flexible schema: Allows for schema-less or flexible schema designs, enabling easier modifications and quicker development cycles.
- High availability: Often designed for high availability and fault tolerance.
- Disadvantages:
- Data consistency: May not always provide strong consistency guarantees, potentially leading to data inconsistencies.
- Query limitations: Complex queries and joins may be more difficult or less efficient.
- Maturity: May have a smaller community and fewer established tools compared to relational databases.
The choice between relational and NoSQL databases depends on the specific requirements of the food delivery business. For example, a platform prioritizing strong data consistency and complex relationships might opt for a relational database. Conversely, a platform that prioritizes scalability and flexibility might choose a NoSQL database. In many cases, a hybrid approach, using both types of databases, can be the most effective solution.
API Endpoints
API endpoints define the entry points for communication between the frontend (user interface, mobile app) and the backend. They allow the frontend to request data, perform actions, and receive responses from the server. Designing well-defined API endpoints is essential for a seamless and efficient user experience. The following are examples of API endpoints required for a food delivery business:
- User Authentication:
POST /users/register
: Registers a new user.POST /users/login
: Logs in an existing user.POST /users/logout
: Logs out the current user.GET /users/me
: Retrieves the current user’s profile.PUT /users/me
: Updates the current user’s profile.POST /users/password/reset
: Initiates a password reset request.POST /users/password/reset/confirm
: Confirms a password reset.- Restaurant Data Retrieval:
GET /restaurants
: Retrieves a list of restaurants (with optional filtering and pagination).GET /restaurants/restaurant_id
: Retrieves details of a specific restaurant.GET /restaurants/restaurant_id/menu
: Retrieves the menu for a specific restaurant.GET /restaurants/search?query=search_term
: Searches for restaurants based on a search term.- Order Management:
POST /orders
: Creates a new order.GET /orders/order_id
: Retrieves details of a specific order.GET /users/me/orders
: Retrieves a list of the user’s orders.PUT /orders/order_id/cancel
: Cancels an order.PUT /orders/order_id/confirm
: Confirms an order (for restaurant).PUT /orders/order_id/prepare
: Marks order as being prepared.PUT /orders/order_id/deliver
: Marks order as being out for delivery.PUT /orders/order_id/complete
: Marks order as delivered.- Delivery Tracking:
GET /delivery_personnel/available
: Retrieves available delivery personnel.PUT /orders/order_id/assign_delivery_personnel
: Assigns a delivery personnel to an order.GET /orders/order_id/tracking
: Retrieves the real-time tracking information for an order.PUT /delivery_personnel/me/location
: Updates the delivery personnel’s current location.
These endpoints allow the frontend to perform key actions such as registering a user, searching for restaurants, placing an order, and tracking delivery. They also provide flexibility for future features.
Data Security Measures
Implementing robust data security measures is paramount for protecting user data, preventing unauthorized access, and ensuring the integrity of the system. The following methods should be incorporated into the backend code:
- Encryption:
- Data at rest: Encrypt sensitive data stored in the database (e.g., passwords, credit card information). Use strong encryption algorithms such as AES-256.
- Data in transit: Use HTTPS/TLS to encrypt all communication between the frontend and the backend.
- Authentication and Authorization:
- Authentication: Implement secure authentication mechanisms, such as password hashing (using bcrypt or Argon2) and multi-factor authentication (MFA).
- Authorization: Implement role-based access control (RBAC) to restrict access to resources based on user roles (e.g., user, restaurant owner, delivery personnel, admin).
- Input Validation:
- Validate all user inputs on the server-side to prevent injection attacks (e.g., SQL injection, cross-site scripting).
- Sanitize inputs to remove or encode potentially harmful characters.
- Regular Security Audits and Updates:
- Conduct regular security audits to identify and address vulnerabilities.
- Keep all software and libraries up-to-date with the latest security patches.
- Rate Limiting and Throttling:
- Implement rate limiting to prevent abuse and denial-of-service (DoS) attacks.
- Throttle requests to protect the server from being overwhelmed.
Restaurant Data Table Schema
The following table schema provides a clear structure for storing restaurant data, which can be adapted based on specific business requirements. This table structure allows for efficient querying and management of restaurant information. The data is structured to be responsive and easily accessible, supporting the core functions of a food delivery service.
Field Name | Data Type | Constraints | Description |
---|---|---|---|
restaurant_id | INT | PRIMARY KEY, AUTO_INCREMENT | Unique identifier for the restaurant. |
restaurant_name | VARCHAR | NOT NULL | Name of the restaurant. |
description | TEXT | Description of the restaurant. | |
cuisine_type | VARCHAR | Type of cuisine offered (e.g., Italian, Chinese). | |
address | TEXT | Restaurant’s address. | |
latitude | DECIMAL | Restaurant’s latitude coordinate. | |
longitude | DECIMAL | Restaurant’s longitude coordinate. | |
phone_number | VARCHAR | Restaurant’s phone number. | |
opening_hours | TEXT | Restaurant’s opening hours (e.g., “10:00-22:00”). | |
image_url | VARCHAR | URL of the restaurant’s image. | |
created_at | TIMESTAMP | Timestamp indicating when the restaurant record was created. | |
updated_at | TIMESTAMP | Timestamp indicating the last time the restaurant record was updated. |
Order Management and Delivery Tracking
Efficient order management and real-time delivery tracking are crucial for the success of a food delivery business. These features directly impact customer satisfaction by providing transparency and ensuring timely delivery. This section details the core components of order management, including matching orders with delivery personnel, calculating estimated delivery times, tracking deliveries, and handling order modifications.
Matching Orders with Delivery Personnel
The process of matching orders with available delivery personnel involves a complex algorithm that considers various factors to optimize efficiency and minimize delivery times. The system must analyze each order and assign it to the most suitable driver.
- Location Proximity: The primary factor is the proximity of the delivery personnel to the restaurant and the customer. The system uses the driver’s current location (obtained via GPS) and the restaurant’s and customer’s addresses to calculate distances, usually using the Haversine formula or similar distance calculations. Drivers closest to the restaurant or customer are prioritized.
- Availability: Only available drivers are considered. Availability status is determined by the driver’s logged-in status, current order assignments, and any pre-scheduled breaks.
- Order Priority: Orders can have different priorities (e.g., based on order type, customer tier, or promised delivery time). High-priority orders are assigned to drivers more quickly. This might involve a weighting system where priority influences the matching score.
- Driver Capacity: Each driver has a capacity limit (e.g., number of orders they can handle simultaneously, or vehicle capacity). The system ensures drivers are not overloaded.
- Order Type: The system considers the order type, such as if the order is for a single customer or for a large group, and the driver’s vehicle capacity.
- Driver Preference: In some systems, drivers can set preferences (e.g., area, order size). These preferences are considered, though they might not always be the deciding factor.
- Algorithm Example: The matching algorithm could use a scoring system, where each factor contributes points. The driver with the highest score for a given order is assigned the order.
Algorithms for Calculating Estimated Delivery Times and Updating Delivery Statuses
Accurate estimation of delivery times and real-time status updates are vital for customer satisfaction. These calculations are dynamic and are continuously refined as new data becomes available.
- Estimated Delivery Time (EDT) Calculation: The EDT is calculated based on several factors:
- Restaurant Preparation Time: The system uses historical data for each restaurant to estimate preparation time. This data is updated based on order size, complexity, and current restaurant load.
- Travel Time: Travel time is estimated using mapping APIs (e.g., Google Maps, Mapbox) that consider traffic conditions, distance, and road types. The algorithm dynamically adjusts the estimated travel time based on real-time traffic data. For example, if there’s heavy traffic on the planned route, the estimated time is increased.
- Driver Travel Time: The travel time from the restaurant to the customer’s location is also factored in.
- Order Pickup Time: This includes the time the driver spends at the restaurant to pick up the order.
- EDT Formula:
EDT = Preparation Time + (Restaurant to Customer Travel Time) + Order Pickup Time
- Real-time Delivery Status Updates: Delivery statuses are updated in real-time based on the driver’s actions and location.
- Order Accepted: When a driver accepts the order.
- Order Picked Up: When the driver marks the order as picked up from the restaurant.
- En Route: When the driver is en route to the customer.
- Arrived: When the driver arrives at the delivery location.
- Delivered: When the order is successfully delivered.
- Real-time Location Tracking: The system continuously tracks the driver’s location using GPS. This data is used to update the delivery status and provide real-time tracking to the customer. The location data is typically transmitted at regular intervals (e.g., every 15-30 seconds).
Integrating with Mapping APIs
Integration with mapping APIs is essential for providing delivery routes and tracking delivery personnel locations. The mapping API provides several functionalities.
- Route Planning: The API is used to calculate the optimal route from the restaurant to the customer’s location. The route calculation considers real-time traffic conditions, road closures, and other factors that may affect travel time.
- Real-time Traffic Data: The API provides real-time traffic data, which is used to adjust estimated delivery times and update routes dynamically. For example, if there is a traffic jam on the planned route, the system can reroute the driver.
- Geocoding: The API is used to convert addresses into geographic coordinates (latitude and longitude), and vice versa. This allows the system to accurately locate the restaurant, customer, and delivery personnel.
- Driver Location Tracking: The API provides the ability to display the driver’s location on a map in real-time. This information is used to provide customers with delivery tracking updates.
- Example: A food delivery business can use the Google Maps API to display the driver’s location on a map, along with the estimated time of arrival (ETA).
Procedures for Handling Order Cancellations, Modifications, and Refunds
Order cancellations, modifications, and refunds are inevitable. Efficiently handling these processes is essential for maintaining customer satisfaction and minimizing financial losses.
- Order Cancellations:
- Before Preparation: If a customer cancels an order before the restaurant begins preparation, the cancellation is usually straightforward, and a full refund is issued.
- During Preparation: If a customer cancels during preparation, the refund policy may vary depending on the restaurant’s policy and the stage of preparation. A partial refund might be issued.
- After Dispatch: Cancellations after the order has been dispatched are generally not allowed. The customer might still be charged for the order.
- Procedure: The system allows customers to cancel orders through the app or website. Cancellation requests are reviewed, and refunds are processed according to the defined policies.
- Order Modifications:
- Before Preparation: Customers can modify their orders (e.g., change items, add instructions) before the restaurant begins preparation.
- During Preparation: Modifications during preparation may or may not be possible, depending on the restaurant’s policy and the type of modification.
- Procedure: The system allows customers to submit modification requests. The requests are forwarded to the restaurant, and the order is updated if the modifications are accepted.
- Refunds:
- Reasons for Refunds: Refunds may be issued for various reasons, including order cancellations, missing items, incorrect items, or delivery issues.
- Refund Processing: Refunds are processed through the payment gateway used for the order. The refund amount is calculated based on the reason for the refund and the order’s value.
- Refund Policy: A clear refund policy is defined and communicated to customers. The policy specifies the conditions under which refunds are issued, the refund amount, and the refund process.
Flow Chart: Order Lifecycle
The order lifecycle, from placement to delivery, is a series of steps that can be visualized using a flowchart. The flowchart illustrates the sequence of events and the transitions between them.
Order Lifecycle Flowchart Description:
The flowchart begins with the customer placing an order. The order then goes to the restaurant. The restaurant accepts the order, and begins preparing it. Concurrently, the system searches for an available driver. Once the order is ready, the driver is notified and picks up the order.
The driver proceeds to the customer’s delivery location. Upon arrival, the order is delivered. The customer receives the order and the delivery is completed. If there is an issue, such as cancellation or a problem with the order, the system initiates a refund process, if applicable.
Payment Processing and Financial Transactions
The integration of robust payment processing capabilities is critical for the success of any food delivery business. This section Artikels the essential components for handling financial transactions, ensuring a seamless and secure experience for both customers and the business. The focus is on integrating with payment gateways, managing transactions, and maintaining financial integrity.
Integrating with Payment Gateways
Integrating with payment gateways such as Stripe and PayPal enables online payment processing. This integration allows customers to securely pay for their orders using various methods, including credit cards, debit cards, and digital wallets. The process involves several key steps.
- API Integration: The first step involves integrating with the payment gateway’s Application Programming Interface (API). This allows your application to communicate with the payment gateway to process transactions.
- Authentication: Securely authenticating with the payment gateway is essential. This usually involves obtaining API keys from the gateway provider and securely storing them in your application’s configuration.
- Payment Forms: Implement payment forms within the user interface to collect payment information securely. These forms should adhere to the payment gateway’s requirements and best practices for security.
- Tokenization: Utilize tokenization to protect sensitive cardholder data. Instead of storing actual card details, the payment gateway provides a token that represents the card. This token is then used for subsequent transactions.
- Webhooks: Implement webhooks to receive real-time notifications from the payment gateway about transaction events, such as successful payments, refunds, and disputes.
Handling Payment Authorization, Capture, and Refunds
Handling payment authorization, capture, and refunds involves a series of code implementations to manage the transaction lifecycle. These code snippets provide examples of how to manage these processes.
- Payment Authorization: When a customer places an order, the application authorizes the payment. This confirms that the customer has sufficient funds but does not immediately transfer the money.
- Payment Capture: After the order is fulfilled (e.g., food is delivered), the payment is captured. This is when the funds are transferred from the customer’s account to the business’s account.
- Refunds: Refunds are processed if there is an issue with the order. The application initiates a refund through the payment gateway, returning the funds to the customer.
Here are example snippets in Python (using Stripe library) to illustrate the processes:
Payment Authorization (Illustrative – Requires a frontend integration to collect card details):
import stripe
stripe.api_key = "YOUR_STRIPE_SECRET_KEY"
try:
payment_intent = stripe.PaymentIntent.create(
amount=1000, # Amount in cents (e.g., $10.00)
currency="usd",
payment_method_types=["card"],
capture_method="manual", # For authorization only
)
print(f"Payment Intent ID: payment_intent.id")
# Store payment_intent.id for later capture or cancellation
except stripe.error.StripeError as e:
print(f"Stripe Error: e")
Payment Capture:
import stripe
stripe.api_key = "YOUR_STRIPE_SECRET_KEY"
try:
payment_intent = stripe.PaymentIntent.capture(
"pi_xxxxxxxxxxxxxxxxxxxxx" # Replace with the Payment Intent ID
)
print("Payment captured successfully")
except stripe.error.StripeError as e:
print(f"Stripe Error: e")
Refund:
import stripe
stripe.api_key = "YOUR_STRIPE_SECRET_KEY"
try:
refund = stripe.Refund.create(
payment_intent="pi_xxxxxxxxxxxxxxxxxxxxx", # Replace with the Payment Intent ID
amount=500, # Amount to refund in cents (e.g., $5.00)
)
print(f"Refund created: refund.id")
except stripe.error.StripeError as e:
print(f"Stripe Error: e")
Generating Invoices and Managing Financial Reports
Generating invoices and managing financial reports are essential for maintaining accurate financial records and providing transparency to customers and stakeholders. The system should automatically generate invoices for each completed order.
- Invoice Generation: Invoices should include details such as order number, date, items ordered, prices, taxes, delivery fees, and the total amount due.
- Invoice Storage: Invoices can be stored in the database and made accessible to both the customer and the business.
- Financial Reporting: The system should generate financial reports, including sales reports, tax reports, and payment summaries. These reports should be customizable based on date ranges and other criteria.
- Integration with Accounting Software: Consider integrating the system with accounting software like QuickBooks or Xero to streamline financial management.
Security Measures for Financial Data Protection
Protecting sensitive financial data is of utmost importance. Several security measures should be implemented to safeguard against data breaches and fraud.
- PCI DSS Compliance: Adhere to the Payment Card Industry Data Security Standard (PCI DSS) if handling credit card information directly. This involves implementing security controls to protect cardholder data.
- Encryption: Encrypt sensitive data, both in transit and at rest. Use HTTPS for secure communication and encrypt data stored in the database.
- Tokenization: Utilize tokenization to replace sensitive cardholder data with tokens. This reduces the risk of exposing card details.
- Regular Security Audits: Conduct regular security audits and penetration testing to identify and address vulnerabilities.
- Fraud Detection: Implement fraud detection mechanisms to identify and prevent fraudulent transactions. This may involve using machine learning algorithms to analyze transaction patterns.
- Secure Storage: Never store sensitive cardholder data on your servers unless absolutely necessary and compliant with PCI DSS. Use payment gateways to handle card details.
Calculating Total Order Amount
The total order amount calculation should accurately reflect the cost of the order, including items, taxes, and delivery fees.
Here’s an example of calculating the total order amount in Python:
def calculate_total(subtotal, tax_rate, delivery_fee): """ Calculates the total order amount. """ tax_amount = subtotal - tax_rate total = subtotal + tax_amount + delivery_fee return total # Example Usage: subtotal = 25.00 tax_rate = 0.07 # 7% tax delivery_fee = 3.00 total_amount = calculate_total(subtotal, tax_rate, delivery_fee) print(f"Total Amount: $total_amount:.2f")
Scaling and Performance Optimization
To ensure a food delivery business code can handle growth, scalability and performance optimization are critical. This involves strategies for managing concurrent users, handling increasing order volumes, and maintaining system responsiveness. Efficiently addressing these aspects guarantees a smooth user experience and the ability to accommodate future expansion.
Optimizing Code for Concurrent Users and Orders, Food delivery business code
Optimizing code for a large number of concurrent users and orders requires a multi-faceted approach focusing on efficiency and resource management. This is achieved through techniques that minimize bottlenecks and maximize the utilization of system resources.
- Asynchronous Processing: Implement asynchronous tasks for non-critical operations such as sending notifications or processing background tasks. This prevents blocking the main thread and improves responsiveness. For example, use message queues like RabbitMQ or Kafka to decouple tasks.
- Connection Pooling: Utilize connection pooling for database interactions. This reduces the overhead of establishing new database connections for each request.
- Efficient Data Structures and Algorithms: Employ efficient data structures and algorithms to minimize processing time. Choosing the right data structures can significantly impact performance. For example, use hash maps for quick lookups instead of linear searches.
- Code Profiling and Optimization: Regularly profile the code to identify performance bottlenecks. Use profiling tools to pinpoint slow-running functions and optimize them. Optimize database queries and avoid unnecessary operations.
- Rate Limiting: Implement rate limiting to prevent abuse and protect the system from being overwhelmed by excessive requests from a single user or IP address. This ensures fair resource allocation.
- Caching: Implement caching mechanisms at different levels (e.g., client-side, server-side, database caching) to reduce the load on the database and speed up response times.
Scaling the Backend Infrastructure
Scaling the backend infrastructure involves strategies to handle increasing traffic and data volume. This can be achieved through horizontal scaling, which involves adding more servers to handle the load, and vertical scaling, which involves upgrading the resources of existing servers.
- Horizontal Scaling: Deploy the application across multiple servers. This allows the system to handle more traffic by distributing the load. Implement a load balancer to distribute incoming requests across the servers.
- Database Scaling: Consider database sharding or replication to handle increased data volume and read/write operations. Database sharding divides the database into smaller parts, while replication creates copies of the database.
- Cloud Infrastructure: Leverage cloud platforms like AWS, Google Cloud, or Azure, which offer scalable resources and services. Use auto-scaling features to automatically adjust resources based on demand.
- Microservices Architecture: Design the backend using a microservices architecture, where different functionalities are implemented as independent services. This allows for independent scaling of individual services.
- Content Delivery Network (CDN): Use a CDN to cache static content (images, CSS, JavaScript) closer to users, reducing latency and improving performance.
Caching Data and Optimizing Database Queries
Caching data and optimizing database queries are crucial for improving performance. Caching stores frequently accessed data in a faster storage medium, while query optimization reduces the time it takes to retrieve data from the database.
- Caching Strategies: Implement various caching strategies, including:
- Client-side caching: Cache static content (images, CSS, JavaScript) in the user’s browser.
- Server-side caching: Cache frequently accessed data on the server (e.g., using Redis or Memcached).
- Database caching: Utilize database-level caching to store query results.
- Query Optimization Techniques: Optimize database queries to improve performance.
- Use indexes: Create indexes on frequently queried columns to speed up data retrieval.
- Optimize JOINs: Ensure efficient use of JOIN operations.
- Avoid SELECT
-: Specify only the required columns in the SELECT statement. - Use query optimization tools: Utilize database-specific tools to analyze and optimize queries.
- Cache Invalidation: Implement mechanisms to invalidate cached data when the underlying data changes. This ensures data consistency.
- Cache-aside pattern: Implement the cache-aside pattern, where the application first checks the cache for data and, if not found, retrieves it from the database and stores it in the cache.
Monitoring System Performance and Identifying Bottlenecks
Monitoring system performance is essential for identifying bottlenecks and ensuring the system operates efficiently. This involves collecting performance metrics, analyzing them, and taking corrective actions.
- Performance Metrics: Collect key performance indicators (KPIs) such as:
- Response time: The time it takes for the server to respond to a request.
- Throughput: The number of requests processed per unit of time.
- Error rates: The percentage of requests that result in errors.
- CPU utilization: The percentage of CPU resources being used.
- Memory utilization: The amount of memory being used.
- Monitoring Tools: Use monitoring tools to collect and analyze performance data.
- Application Performance Monitoring (APM) tools: Tools like New Relic, Datadog, or Prometheus can monitor application performance and identify bottlenecks.
- Server monitoring tools: Tools like Nagios or Zabbix can monitor server health and resource usage.
- Database monitoring tools: Tools like pgAdmin or MySQL Workbench can monitor database performance.
- Alerting and Notifications: Set up alerts to be notified when performance metrics exceed predefined thresholds.
- Regular Performance Reviews: Conduct regular performance reviews to identify and address performance issues.
Implementing Load Balancing
Implementing load balancing is a key strategy for distributing traffic across multiple servers. This ensures that no single server is overwhelmed and that the system remains responsive even under heavy load.
- Load Balancer Types: Choose an appropriate load balancer based on the requirements.
- Hardware Load Balancers: Dedicated hardware devices designed for load balancing (e.g., F5 BIG-IP).
- Software Load Balancers: Software-based solutions that run on servers (e.g., HAProxy, Nginx).
- Cloud-based Load Balancers: Load balancers provided by cloud platforms (e.g., AWS Elastic Load Balancing, Google Cloud Load Balancing).
- Load Balancing Algorithms: Implement load balancing algorithms to distribute traffic effectively.
- Round Robin: Distributes requests sequentially to each server.
- Least Connections: Directs requests to the server with the fewest active connections.
- IP Hash: Directs requests from the same IP address to the same server.
- Health Checks: Configure health checks to monitor the health of the servers. The load balancer will only forward traffic to healthy servers.
- Session Persistence: Implement session persistence to ensure that users are directed to the same server for the duration of their session.
- SSL Termination: Terminate SSL connections at the load balancer to offload the encryption and decryption processing from the application servers.
Code Security and Best Practices
The security of a food delivery business’s code is paramount. Vulnerabilities can lead to data breaches, financial losses, and reputational damage. Implementing robust security measures throughout the development lifecycle is crucial to protect both the business and its users. This section details common vulnerabilities, prevention methods, and best practices for secure coding in the context of a food delivery platform.
Common Security Vulnerabilities
Food delivery applications are susceptible to various security threats. Understanding these vulnerabilities is the first step towards building a secure platform.
- SQL Injection: This occurs when malicious SQL code is injected into input fields, potentially allowing attackers to access, modify, or delete data in the database. For example, an attacker could manipulate the order history or user credentials.
- Cross-Site Scripting (XSS): XSS attacks inject malicious scripts into websites viewed by other users. Attackers can steal user session cookies, redirect users to phishing sites, or deface the website. For instance, an attacker might inject a script into a restaurant review to steal user data.
- Cross-Site Request Forgery (CSRF): CSRF attacks trick users into performing unwanted actions on a web application where they are authenticated. This can lead to unauthorized fund transfers or changes to user accounts. An example would be an attacker tricking a user into changing their delivery address.
- Broken Authentication and Session Management: Weak authentication mechanisms or poor session management can allow attackers to gain unauthorized access to user accounts. This includes weak password policies, lack of multi-factor authentication (MFA), and insecure session cookie handling.
- Insecure Direct Object References: This vulnerability arises when an application directly exposes internal object references, such as database keys or filenames. Attackers can exploit this to access or modify sensitive data. For example, an attacker might change the order status of other users.
- Security Misconfiguration: Incorrectly configured servers, frameworks, and databases can create security holes. This includes leaving default credentials unchanged, failing to update software, and misconfiguring access controls.
- Insecure Deserialization: If an application deserializes user-controlled data without proper validation, it can lead to remote code execution. Attackers can inject malicious code during the deserialization process.
- Using Components with Known Vulnerabilities: Relying on outdated or vulnerable third-party libraries and frameworks can introduce security risks. Regular updates and vulnerability scanning are essential.
Preventing Security Vulnerabilities Through Secure Coding Practices
Preventing security vulnerabilities requires a proactive approach to coding. This involves adopting secure coding practices throughout the development process.
- Input Validation: Validate all user inputs on both the client-side and server-side to prevent SQL injection, XSS, and other injection attacks. Use parameterized queries or prepared statements to prevent SQL injection.
- Output Encoding: Encode output to prevent XSS attacks. This involves escaping special characters in the output based on the context where the data is displayed (e.g., HTML, JavaScript, URL).
- Implement CSRF Protection: Use CSRF tokens to prevent CSRF attacks. These tokens are unique, secret values generated by the server and included in forms and requests.
- Secure Authentication and Session Management: Implement strong password policies, multi-factor authentication (MFA), and secure session cookie handling (e.g., HTTPOnly, Secure flags). Regularly review and update authentication mechanisms.
- Principle of Least Privilege: Grant users and processes only the minimum necessary permissions. This limits the impact of a potential security breach.
- Regular Security Audits and Penetration Testing: Conduct regular security audits and penetration testing to identify and address vulnerabilities. These tests simulate real-world attacks to assess the security posture of the application.
- Keep Software Up-to-Date: Regularly update all software components, including operating systems, frameworks, libraries, and databases, to patch known vulnerabilities.
- Use Security Headers: Configure security headers (e.g., Content Security Policy, X-Frame-Options) to mitigate various types of attacks.
- Error Handling and Logging: Implement robust error handling and logging to detect and respond to security incidents. Avoid displaying sensitive information in error messages.
Implementing User Authentication and Authorization Mechanisms
Secure user authentication and authorization are fundamental to protecting user accounts and data.
Discover how www.always food safe.com has transformed methods in this topic.
- Authentication: Implement a secure authentication process, such as using strong password hashing algorithms (e.g., bcrypt, Argon2) and salting. Consider using MFA for enhanced security.
- Authorization: Implement role-based access control (RBAC) to define user roles and permissions. This ensures that users can only access the resources and perform the actions they are authorized to.
- Session Management: Securely manage user sessions by using session cookies with appropriate flags (e.g., HTTPOnly, Secure) and implementing session timeouts.
- API Authentication: Securely authenticate API requests using methods such as API keys, OAuth, or JWT (JSON Web Tokens).
- Rate Limiting: Implement rate limiting to prevent brute-force attacks and denial-of-service (DoS) attacks.
Protecting Sensitive Data
Protecting sensitive data, such as passwords and payment information, is crucial for maintaining user trust and complying with data privacy regulations.
- Password Protection: Always hash and salt passwords using strong algorithms. Never store passwords in plain text.
- Payment Information Security: Handle payment information securely by using PCI DSS compliant payment gateways and encrypting sensitive data. Never store credit card details directly.
- Data Encryption: Encrypt sensitive data both in transit (e.g., using HTTPS) and at rest (e.g., using database encryption).
- Data Minimization: Collect and store only the necessary data. Delete data when it is no longer needed.
- Secure Storage: Store sensitive data in secure locations, such as encrypted databases or secure vaults.
Code Review Checklist for Security and Quality
A code review checklist helps ensure code quality and security.
- Input Validation: Verify that all user inputs are properly validated to prevent injection attacks.
- Output Encoding: Check for proper output encoding to prevent XSS attacks.
- Authentication and Authorization: Review the authentication and authorization mechanisms for security and correctness.
- Session Management: Examine the session management implementation for security flaws.
- Data Handling: Ensure that sensitive data is handled securely, including encryption and proper storage.
- Error Handling and Logging: Verify that error handling and logging are implemented correctly.
- Third-Party Libraries: Check for the use of outdated or vulnerable third-party libraries.
- Code Style and Conventions: Ensure that the code adheres to established coding standards and best practices.
- Testing: Review the test coverage and ensure that adequate tests are in place.
- Security Headers: Confirm that security headers are properly configured.
Testing and Deployment
Effective testing and deployment are critical for the success of a food delivery business code. Rigorous testing ensures the code functions as expected, while a well-defined deployment process minimizes downtime and ensures a smooth transition to the production environment. This section details the different types of testing, deployment procedures, and monitoring strategies essential for a reliable and scalable food delivery platform.
Types of Testing
Thorough testing is essential to identify and fix bugs, ensuring a stable and reliable application. Several testing methodologies should be incorporated throughout the development lifecycle.
- Unit Tests: Unit tests verify the functionality of individual code modules or components in isolation. They ensure that each unit of code behaves as intended. For example, a unit test might check if a function correctly calculates the total price of an order based on the items selected and their quantities.
- Integration Tests: Integration tests verify the interaction between different modules or components of the application. They ensure that the integrated parts work correctly together. For instance, an integration test would verify that the order placement module correctly interacts with the payment processing module and the database.
- User Acceptance Tests (UAT): User Acceptance Tests are performed by the end-users or stakeholders to validate that the system meets the business requirements and is ready for deployment. This involves testing the application from the user’s perspective, focusing on the overall functionality and usability. UAT ensures that the system meets the needs of both customers and restaurant partners.
- Performance Tests: Performance tests measure the application’s response time, throughput, and resource usage under various load conditions. These tests help to identify performance bottlenecks and ensure the application can handle the expected user traffic. Load testing and stress testing are examples of performance testing.
- Security Tests: Security tests are conducted to identify vulnerabilities in the application that could be exploited by malicious actors. These tests include penetration testing, vulnerability scanning, and code reviews to ensure the application is secure and protected against common threats.
Writing and Executing Unit Tests
Unit tests are a fundamental part of software development, allowing developers to verify the functionality of individual code components. They should be written early and often throughout the development process.
- Choosing a Testing Framework: Select a suitable testing framework for the programming language used (e.g., JUnit for Java, pytest for Python, or Jest for JavaScript).
- Test Case Design: Create test cases that cover various scenarios, including positive and negative test cases, edge cases, and boundary conditions. Positive test cases verify the expected behavior, while negative test cases test how the code handles unexpected inputs or errors.
- Test Structure: Organize tests logically, typically using a “Arrange-Act-Assert” pattern:
- Arrange: Set up the necessary preconditions for the test (e.g., initializing objects, setting input data).
- Act: Execute the code being tested.
- Assert: Verify the results against the expected outcomes.
- Test Execution: Execute the unit tests frequently, ideally automatically as part of the build process.
- Example (Python with pytest):
Consider a function to calculate the total price of items:
def calculate_total(items):
total = 0
for item in items:
total += item['price']
- item['quantity']
return total
A unit test might look like this:
import pytest
from your_module import calculate_totaldef test_calculate_total():
items = [
'price': 10, 'quantity': 2,
'price': 5, 'quantity': 3
]
assert calculate_total(items) == 35
This test case defines a set of items, calculates the total price using the `calculate_total` function, and then asserts that the returned value is equal to the expected total.
Deployment Procedures
Deploying the code to a production environment involves several steps to ensure a smooth and reliable transition. The specific procedures will vary depending on the chosen technologies and infrastructure.
- Code Compilation: Compile the source code into executable files or bytecode, depending on the programming language and platform.
- Database Migration: Apply any necessary database schema changes or data migrations. This ensures that the database structure is up-to-date with the latest code changes.
- Server Configuration: Configure the servers to host the application, including setting up the necessary software, dependencies, and environment variables.
- Code Deployment: Deploy the compiled code and any associated assets (e.g., images, CSS files) to the servers.
- Testing: Conduct post-deployment testing to verify that the application is functioning correctly in the production environment.
- Rollback Strategy: Have a rollback strategy in place in case any issues arise after deployment. This involves being able to quickly revert to a previous, stable version of the code.
Monitoring the System After Deployment
After deployment, continuous monitoring is crucial to identify and resolve any issues that may arise. Monitoring helps ensure the application’s stability, performance, and security.
- Application Performance Monitoring (APM): Use APM tools to track key performance indicators (KPIs) such as response times, error rates, and resource utilization. Examples include Prometheus, Grafana, New Relic, and Datadog.
- Error Tracking: Implement error tracking to capture and analyze application errors. This allows for quick identification and resolution of bugs. Tools like Sentry and Bugsnag can be used.
- Log Analysis: Analyze application logs to identify potential issues, security threats, and performance bottlenecks. Log aggregation and analysis tools like the ELK stack (Elasticsearch, Logstash, Kibana) or Splunk are commonly used.
- Alerting: Set up alerts to be notified of critical events, such as high error rates, server outages, or security breaches.
- User Feedback: Collect user feedback to identify usability issues, bugs, or areas for improvement. This can be done through surveys, feedback forms, or direct communication channels.
- Regular Audits: Conduct regular security audits and code reviews to ensure the system remains secure and compliant with industry best practices.
Deployment Process Steps
The deployment process involves a series of steps that must be followed to ensure the application is successfully deployed to the production environment.
- Code Compilation and Build: Compile the source code and create an executable package or artifact.
- Database Migration: Apply any necessary database schema changes using tools like Flyway or Liquibase.
- Server Configuration: Configure the servers to host the application, including setting up the environment, dependencies, and any required services.
- Code Deployment: Deploy the compiled code and any necessary assets to the servers. This can be done manually or using automated deployment tools like Jenkins, GitLab CI, or CircleCI.
- Testing and Validation: Conduct post-deployment testing to verify that the application is functioning correctly.
- Monitoring and Alerting: Implement monitoring and alerting to track the application’s performance and identify any issues.
- Rollback (if necessary): Have a rollback strategy in place to revert to a previous, stable version of the code in case of issues.
Wrap-Up
In conclusion, mastering food delivery business code requires a multifaceted approach, blending technical expertise with a keen understanding of user experience and business requirements. This comprehensive guide provides the essential building blocks for creating a thriving food delivery platform. By focusing on core components, architectural best practices, and a commitment to security and performance, developers can build solutions that meet the evolving demands of this dynamic market.